Configuring Java
With OpenL you can built a language
configuration that looks and feels like Java (any Java programmer would feel
comfortable with it from the moment she sees it) but that overcomes well-known
Java limitations (you
can find interesting critiques of Java, C# and other similar languages
at
http://www.research.microsoft.com and
http://www.jwz.org/doc/java.html). OpenL-based Java configuration “openl.j” includes
such additional features like operator overloading allowing you to write the
arithmetic expressions in a natural way understandable to a non-technical
specialists. For example, you do not want to offer your users a funny looking
code like x.add(y.abs().mul(z)) instead of
the intuitive x+|y|*z.
If a user wants to tell that
customer.mothlyIncome<3*loan.amount/loan.term
it should be exactly what you allow her to write instead of something
strange like
customer.getMothlyIncome().less( 3*loan.getAmount().div(loan.getTerm()))
You may also define operators that allow you to add miles to kilometers
and present results in meters. So, the code like X[mi]+Y[km] or
5[h]+25[min]+30[s] presents valid OpenL expressions. You may download
and analyze examples from here.
With OpenL you may also configure your Java in a way that
limits some Java features (like an access to a file system) on the
language level for the security purposes. If your application accepts a code
from a remote machine, you do not want to jeopardize your computer and rely
just on security features built-in into Internet Explorer. If you
configure OpenL in the way that does not allow system calls, the code with
such calls will be syntactically incorrect that will prevent it from
execution.
Java Snippets
When it comes to
representation of serious business logic, in most cases people still end up
with languages like Java or C#. After all marketing mantras about a natural
"English-like" language, you still need a real programming
language with power and expressiveness of Java. But at some point, you want
to present the business
logic in a way
that can be supported by non-programmers. So, you actually want
to open Java by distributing your business logic between
different placeholders:
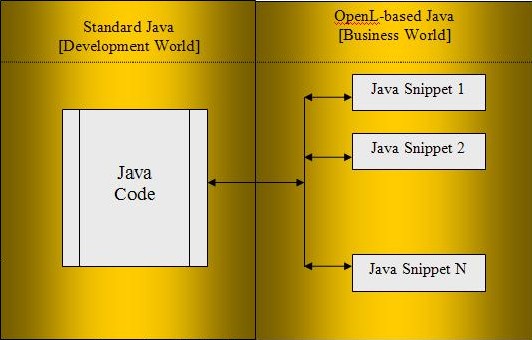
OpenL opens Java supporting Java Snippets for
business use outside of traditional software development environment. For example, Excel-based
decision tables oriented to business people can contain complex business
rules whose implementation requires small pieces of Java code presented in
the same Excel spreadsheet but probably hidden from a business user. See
examples at
http://openrules.com/examples.htm.
Along with the standard Java you can
present logic of your business functions in Java Snippets written in “openl.j”.
These snippets can use directly all Java objects defined in your
application. All standard or special packages can also be used directly
from Java snippets like from a regular Java.
The snippets can be kept in a separate
file as a fragment of a Java code like in this example:
// openl
int
hour =
Calendar.getInstance().get(Calendar.HOUR_OF_DAY);
String
greeting;
if
(hour >
0 &&
hour <=
12)
greeting
= "Good
Morning";
else
if
(hour >
12 &&
hour <=
18)
greeting
= "Good
Afternoon";
else
if
(hour >
18 &&
hour <=
21)
greeting
= "Good
Evening";
else
if
(hour >
21 &&
hour <=
24)
greeting
= "Good
Night";
System.out.println(greeting+",
World!");
Note that
this snippet is using the standard Java class Calendar. Thus, with OpenL,
it is up to a developer to decide which business logic to keep in Java and
which put in the snippets, that can be supported by non-technical or less
technical users outside of a selected Java development environment.
You may
define methods inside snippets and call them from a real Java code or from
other snippets. Here are two snippets with methods:
String
defineCurrentGreeting()
{
int
hour =
Calendar.getInstance().get(Calendar.HOUR_OF_DAY);
String
greeting =
"?";
if
(hour >
0 &&
hour <=
12)
greeting
= "Good
Morning";
else
if
(hour >
12 &&
hour <=
18)
greeting
= "Good
Afternoon";
else
if
(hour >
18 &&
hour <=
21)
greeting
= "Good
Evening";
else
if
(hour >
21 &&
hour <=
24)
greeting
= "Good
Night";
return
greeting;
}
String
definePrefix(Customer
customer)
{
String
prefix;
if
(customer.getGender().equals("M"))
prefix
= "Mr.";
else
{
if
(customer.getMaritalStatus().equals("Married"))
prefix
= "Mrs.";
else
prefix
= "Ms.";
}
return
prefix;
}
This
following snippet will create a customer using the proper Java class
Customer. It uses two previous snippets to salute her with a greeting like
“Good Afternoon, Mrs. Robinson!”:
int
main(String[]
args)
{
String
greeting =
defineCurrentGreeting();
Customer
customer =
defineCustomer();
String
prefix =
definePrefix(customer);
System.out.println(greeting+",
"+prefix+customer.getName()+"!");
return
0;
}
Customer
defineCustomer()
{
Customer
customer =
new
Customer();
customer.setName("Robinson");
customer.setGender("F");
customer.setMaritalStatus("Married");
return
customer;
}
The snippets can be called directly from
your Java code like in this example:
// This string contains a simple OpenL code the same as a regular Java
String
openLcode =
"System.out.println(\"Hello, World!\")";
// Get an OpenL language configuration named "org.openl.j"
OpenL
language =
OpenL.getInstance("org.openl.j");
//evaluate code, see result on console
language.evaluate(new
StringSourceCodeModule(openLcode,
null));
You can present Java snippets in regular
flat files using a Notepad or your favorite text editor. You can manage
large Java projects with snippets using
Eclipse with the provided OpenL's plugin. In this case, Eclipse will
show you all errors in the snippets. When snippets are defined in an Excel
file, double-click to an error will open the Excel with the erroneous cell
selected. You can configure OpenL in different ways to use your
preferable repositories of Java snippets such as XML file, Excel’s
spreadsheets, or MS Word documents.
Access To Different Data
Sources
OpenL provides a direct access to
objects defined in different sources such as Java, MS Office files, DB, XML
including XML Schema, WSDL, RDF, etc. You may use familiar Java syntax to
deal with objects defined in an XML file, in an Excel spreadsheet, or in an
SQL table as if they were a described in a Java class without necessity to
create such classes. No intermediate Java classes are generated either.
Moreover, the same OpenL code can naturally work with objects coming from
different object sources without knowing their origin. For example, in the
snippets above a Customer was defines directly in the OpenL snippet using a
method "defineCustomer". Below are three other ways to define a Customer: